Dialog Controls
These are special controls which provide an interface for displaying a list of values too choose from or for entering of new values, we have 5 dialog controls like ColorDialog, FolderBrowserDialog, FontDialog, OpenFileDialog and SaveFileDialog. Dialog controls are not shown directly on the form even after adding them, we can see them at bottom of the studio in design time, to make them visible in runtime we need to explicitly call the method ShowDialog() on the controls object, which returns a value of type DialogResult (enum), using it we can find out which button has been clicked on the DialogBox like Ok button or Cancel button etc.
Dialog Controls never performs any action they are only responsible for returning the values to application developers that has been chosen by end users or entered by the end users, where the developers are responsible for capturing the values and then perform the necessary actions. To capture the values that are chosen or entered by end users we are provided with following properties
Design a new form as below, add the ColorDialog, FontDialog, OpenFileDialog and SaveFileDialog controls to the form and write the below code:
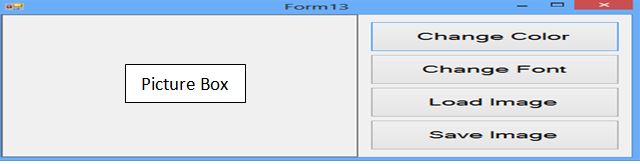
Code under Change Color Button
DialogResult dr = colorDialog1.ShowDialog(); if(dr == DialogResult.OK) { button1.BackColor = colorDialog1.Color; }
Code under Change Font Button
DialogResult dr = fontDialog1.ShowDialog(); if(dr == DialogResult.OK) { button2.Font = fontDialog1.Font; }
openFileDialog1.Filter = "Image Files (*.jpg)|*.jpg|Icon Images (*.ico)|*.ico|All Files (*.*)|*.*"; openFileDialog1.FileName = ""; DialogResult dr = openFileDialog1.ShowDialog(); if (dr != DialogResult.Cancel) { string imgPath = openFileDialog1.FileName; pictureBox1.ImageLocation = imgPath; }
Code under Save Image Button
saveFileDialog1.Filter = "Image Files (*.jpg)|*.jpg|Icon Images (*.ico)|*.ico|All Files (*.*)|*.*"; DialogResult dr = saveFileDialog1.ShowDialog(); if (dr != DialogResult.Cancel) { string imgPath = saveFileDialog1.FileName; pictureBox1.Image.Save(imgPath); }