Recursion
Recursion is a programming technique that allows the programmer to express operations in terms of themselves. Simply we can say that
when a 'c' function calls itself.
In other words recursion is thus the process of defining something in terms of itself.
Recursive functions
void recurse() { recurse(); /* Function calls itself */ } void main() { recurse(); /* Sets off the recursion */ getch(); }
We want to calculate the factorial value using recursive function.
Example of recursion.#include<stdio.h> #include<conio.h> void main( ) { int num, factorial ; clrscr(); printf ( "\nEnter any number " ) ; scanf ( "%d", &num ) ; factorial = fact ( num ) ; printf ( "Factorial value = %d", factorial ) ; getch(); } fact ( int n ) { if ( n == 0 ) return ( 1 ) ; else return(n * fact ( n - 1 ) ; }
Output
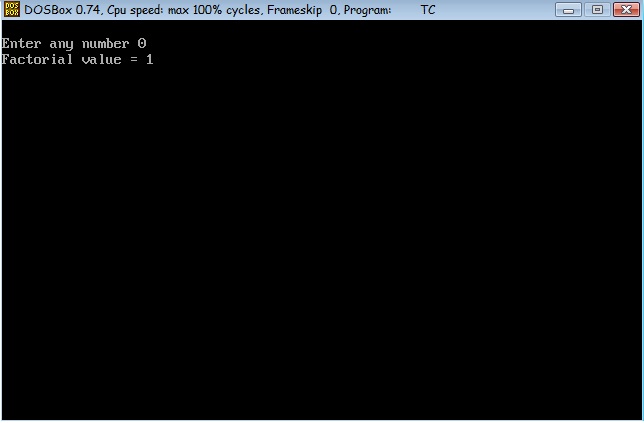
Explaination
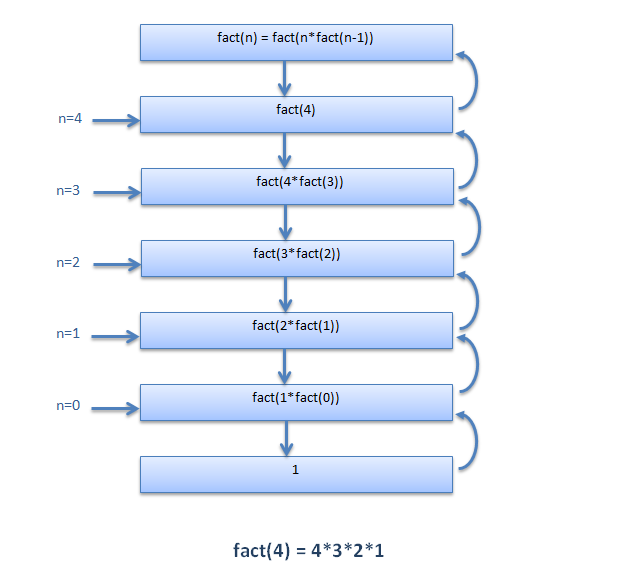