Insertion In Linked list
There are three situation for inserting element in list.
- Insertion at the front of list.
- Insertion in the middle of the list.
- Insertion at the end of the list.
Procedure For Inserting an element to linked list
Step-1: Get the value for NEW node to be added to the list and its position.Step-2: Create a NEW, empty node by calling malloc(). If malloc() returns no error then go to step-3 or else say "Memory shortage".
Step-3: insert the data value inside the NEW node's data field.
Step-4: Add this NEW node at the desired position (pointed by the "location") in the LIST.
Step-5: Go to step-1 till you have more values to be added to the LIST.
Insertion Node In Linked List
void insert(node *ptr, int data) { /* Iterate through the list till we encounter the last node.*/ while(ptr->next!=NULL) { ptr = ptr -> next; } /* Allocate memory for the new node and put data in it.*/ ptr->next = (node *)malloc(sizeof(node)); ptr = ptr->next; ptr->data = data; ptr->next = NULL; }
Insertion at the front of list
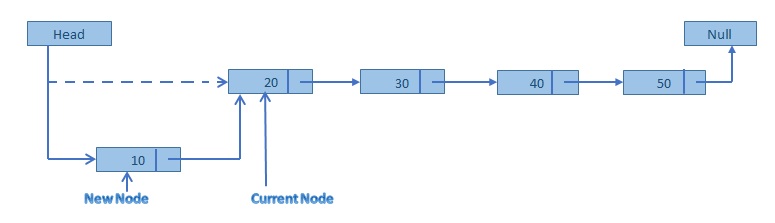
Insertion Node in given location Linked List
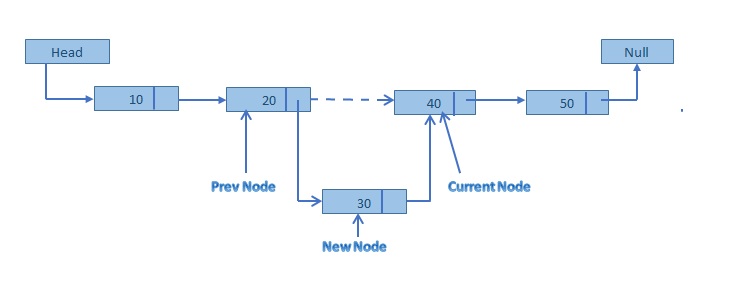
Insertion at the end of the list.
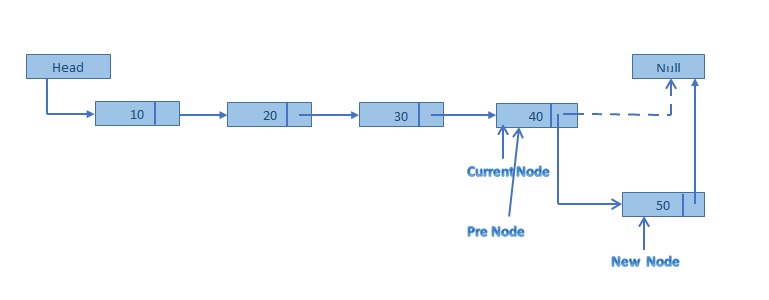