Insertion At Location in doubly linked list
Algorithm
InsertAtlocDll(info,prev,next,start,end,loc,size) 1.set nloc = loc-1 , n=1 2.create a new node and address in assigned to ptr. 3.check[overflow] if(ptr=NULL) write:overflow and exit 4.set Info[ptr]=item; 5.if(start=NULL) set prev[ptr] = next[ptr] = NULL set start = end = ptr else if(nloc<=size) repeat steps a and b while(n != nloc) a. loc = next[loc] b. n = n+1 [end while] next[ptr] = next[loc] prev[ptr] = loc prev[next[loc]] = ptr next[loc] = ptr else set prev[ptr] = end next[end] = ptr set ptr[next] = NULL set end = ptr [end if] 6.Exit.
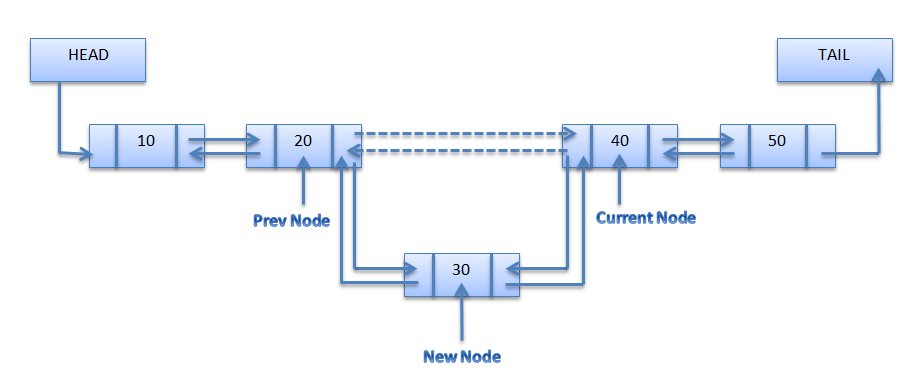
Fuction For Insert at Location
void insertAtloc(node **start,node **end,int item , int i,int size ) { node *ptr,*loc; int n=1 ; i=i-1; ptr=(node*)malloc(sizeof(node)); ptr->info=item; loc = *start ; if(*start==NULL) { ptr->prev = ptr->next = NULL ; *start = *end = ptr ; } else if(i<=size) { while(n != i) { loc=loc->next; n++; } ptr->next = loc->next ; ptr->prev = loc ; (loc->next)->prev = ptr ; loc->next = ptr ; } else { ptr->prev = *end; (*end)->next = ptr ; ptr->next= NULL; (*end)=ptr; } }
C programe for insertion at location in dubly linked list
#include<stdio.h> #include<malloc.h> #include<conio.h> typedef struct Node { struct Node *prev; int info ; struct Node *next; }node; void createdub(node**,node**,int); void insertAtloc(node **,node**,int,int,int); void display(node *); void main() { int ch, item, pos,loc,i; node *start ,*end ; start = end = NULL; clrscr(); printf("Enter number of node: "); scanf("%d",&i); createdub(&start,&end,i); printf("\nThe list is : "); display(start); printf("\nEnter the loc : "); scanf("%d",&loc); printf("\n\nEnter the item to be inserted at loc : "); scanf("%d",&item); insertAtloc(&start,&end,item,loc,i); printf("\nNow the list is : "); display(start); getch(); } void createdub(node **start,node **end,int i) { int item ,k=1; while(i) { node *ptr; printf("\nEnter the info for node %d : ",k); scanf("%d",&item); ptr=(node*)malloc(sizeof(node)); ptr->info=item; if(*start==NULL) { ptr->prev = ptr->next = NULL ; *start = *end = ptr ; } else { ptr->prev = *end; (*end)->next = ptr ; ptr->next= NULL; (*end)=ptr; } i--; k++; } } void insertAtloc(node **start,node **end,int item , int i,int k ) { node *ptr,*loc; int n=1 ; i=i-1; ptr=(node*)malloc(sizeof(node)); ptr->info=item; loc = *start ; if(*start==NULL) { ptr->prev = ptr->next = NULL ; *start = *end = ptr ; } else if(i<=k) { while(n != i) { loc=loc->next; n++; } ptr->next = loc->next ; ptr->prev = loc ; (loc->next)->prev = ptr ; loc->next = ptr ; } else { ptr->prev = *end; (*end)->next = ptr ; ptr->next= NULL; (*end)=ptr; } } void display(node *start) { while(start !=NULL) { printf("\t %d",start->info); start = start->next; } }
Output
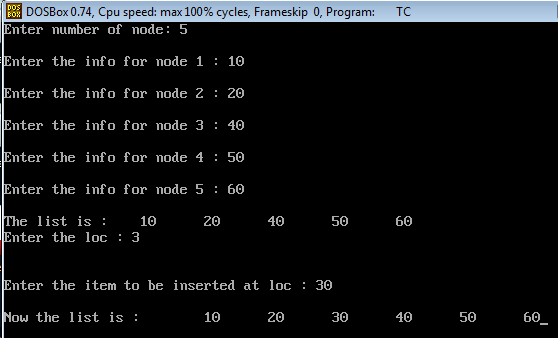